c++에는 c언어와는 다르게 클래스의 외부에서 멤버가 접근하는 권한을 부여하는 접근 지정자를 사용한다. C++은 public, private, protected 세 가지 접근 지정자를 제공한다. public은 공개 멤버이므로 클래스 외부에서도 접근할 수 있고, private는 비공개 멤버이므로 클래스 내에서만 접근할 수 있다. protected는 외부에서는 접근할 수 없으나, 상속된 자식 class에서 접근할 수 있다. 클래스 멤버는 기본적으로 private이지만, public 접근지정자를 사용해서 공개할 수 있다.
일반적으로 멤버변수는 비공개로 하고, 멤버 함수는 공개하는 것이 일반적이다.
1. Class 헤더파일에 private멤버와 public 멤버 선언 및 정의
#pragma once
#include<iostream>
using namespace std;
class motherClass
{
private: //클래스 내에서만 접근 가능
int _day;
int _month;
int _year;
int arr[5] = { 1,2,3,4,5 };
const char* _name = "2023-10-30";
public:
motherClass()//클래스 멤버변수 초기화
:_day(30), _month(10), _year(2023)
{
cout << "클래스 함수 호출" << endl;
}
void Output() { //공개 권한 멤버함수 정의
cout << _year << "년" << _month << "월" << _day << "일" << endl;
cout << _name << endl;
for (int i : arr) { //순환 호출을 통한 배열 출력
cout << i;
}
}
// private 멤버 변수에 접근하기 위해서는 함수 이용
int getday() { return _day; }
void setday(int day) { _day = day; }
const char* getname() { return _name; }
void setname(const char* name) { _name = name; }
};
2. main 함수에서 private과 public 함수 호출 test
#include<iostream>
#include "motherClass.h"
using namespace std;
int main() {
motherClass mo;
mo.Output();
mo.setday(mo.getday() + 1); //private 변수 day 1증가 (main함수에서 바로 접근 불가능)
mo.setname("2023-10-31"); //prive 변수 name 변경 (main 함수에서 바로 접근 불가능)
mo.Output();
}
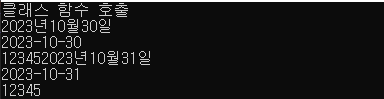
3. protected 멤버 변수
부모클래스를 상속하는 자식클래스가 상속될때 public과 proteced 멤버에 대한 접근 권한을 상속받는다. protected 멤버변수에 대한 테스트는 아래와 같다.
1) classMother의 헤더파일에 protected 형식지정자 해당 멤버변수 추가
#pragma once
#include<iostream>
using namespace std;
class motherClass
{
private: //클래스 내에서만 접근 가능
int _day;
int _month;
int _year;
int arr[5] = { 1,2,3,4,5 };
const char* _name = "2023-10-30";
protected:
int dollarUSD = 1350;
int interest = 5;
public:
motherClass()//클래스 멤버변수 초기화
:_day(30), _month(10), _year(2023)
{
cout << "부모클래스 생성자" << endl;
}
void Output() { //공개 권한 멤버함수 정의
cout << _year << "년" << _month << "월" << _day << "일" << endl;
cout << _name << endl;
for (int i : arr) { //순환 호출을 통한 배열 출력
cout << i;
}
cout << endl;
cout << dollarUSD <<"원"<< endl;
cout << interest <<"%"<< endl;
cout << endl;
}
// private 멤버 변수에 접근하기 위해서는 함수 이용
int getday() { return _day; }
void setday(int day) { _day = day; }
const char* getname() { return _name; }
void setname(const char* name) { _name = name; }
};
2) classMother의 자식 클래스 생성
#pragma once
#include<iostream>
#include "motherClass.h" //부모클래스 include
using namespace std;
class childClass : public motherClass { //부모클래스를 상속하는 자식클래스 생성 public, protected 접근 지정 멤버까지 상속 및 접근권한 부여
public:
childClass()
{
cout << "자식클래스 생성자" << endl;
dollarUSD = 1358;
interest = 5.1;
}
void Output() {
cout << dollarUSD << "원" << endl;
cout << interest << "%" << endl;
}
};
3) main 함수에서 자식클래스와 부모클래스 헤더파일 include 및 함수 호출
#include<iostream>
#include "childClass.h"
using namespace std;
int main() {
motherClass mo;
childClass cc;
mo.Output();
cc.Output();
}
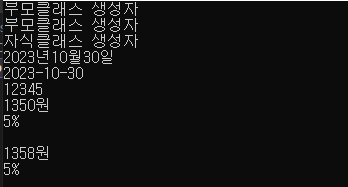